mirror of
https://github.com/MHSanaei/3x-ui.git
synced 2025-03-01 01:20:49 +03:00
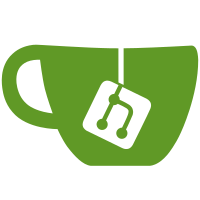
* [refactor] api controller * [fix] access log path better to not hardcode the access log path, maybe some ppl dont want to use the default ./access.log * [fix] set select options from logs paths in xray settings * [update] .gitignore * [lint] all .go files * [update] use status code for jsonMsg and 401 to unauthorize * [update] handle response status code via axios * [fix] set correct value if log paths is set to 'none' we also use the default value for the paths if its set to none * [fix] iplimit - only warning access log if f2b is installed
47 lines
826 B
Go
47 lines
826 B
Go
package random
|
|
|
|
import (
|
|
"math/rand"
|
|
)
|
|
|
|
var (
|
|
numSeq [10]rune
|
|
lowerSeq [26]rune
|
|
upperSeq [26]rune
|
|
numLowerSeq [36]rune
|
|
numUpperSeq [36]rune
|
|
allSeq [62]rune
|
|
)
|
|
|
|
func init() {
|
|
for i := 0; i < 10; i++ {
|
|
numSeq[i] = rune('0' + i)
|
|
}
|
|
for i := 0; i < 26; i++ {
|
|
lowerSeq[i] = rune('a' + i)
|
|
upperSeq[i] = rune('A' + i)
|
|
}
|
|
|
|
copy(numLowerSeq[:], numSeq[:])
|
|
copy(numLowerSeq[len(numSeq):], lowerSeq[:])
|
|
|
|
copy(numUpperSeq[:], numSeq[:])
|
|
copy(numUpperSeq[len(numSeq):], upperSeq[:])
|
|
|
|
copy(allSeq[:], numSeq[:])
|
|
copy(allSeq[len(numSeq):], lowerSeq[:])
|
|
copy(allSeq[len(numSeq)+len(lowerSeq):], upperSeq[:])
|
|
}
|
|
|
|
func Seq(n int) string {
|
|
runes := make([]rune, n)
|
|
for i := 0; i < n; i++ {
|
|
runes[i] = allSeq[rand.Intn(len(allSeq))]
|
|
}
|
|
return string(runes)
|
|
}
|
|
|
|
func Num(n int) int {
|
|
return rand.Intn(n)
|
|
}
|